Create your own WORDLE using Svelte!
May 31, 2022
technicalsveltefunDIWhy
We will be learning how to make a Wordle game using Svelte!
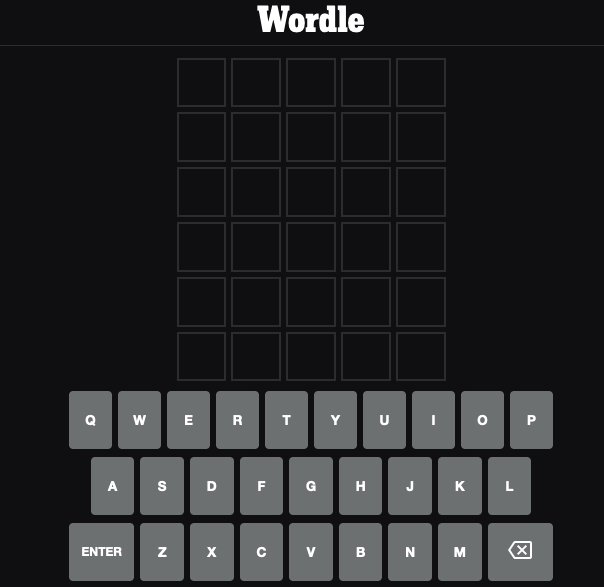
Well now that the craze is slowly coming down for Wordle. I thought it would be fun to create my own version to learn more about Svelte.
I created a Svelte page and started with the basics. I designed the on screen keyboard and a suitable npm package that contains getting random words that are five letters.
import randomWords from "random-words";
const rowOne = ["q", "w", "e", "r", "t", "y", "u", "i", "o", "p"];
const rowTwo = ["a", "s", "d", "f", "g", "h", "j", "k", "l"];
const rowThree = ["bksp", "z", "x", "c", "v", "b", "n", "m", "entr"];
From here I created the “boxes” that each letter would be in and need to display the letters and be able to update boxes on key presses. This is some of the code written for that:
function clickedButton(letter) {
if (letter != "entr") {
if (typed.length <= 4) {
if (letter != "bksp") {
typed[typed.length] = letter;
} else {
if (letter.length > 0) {
typed.pop();
typed = typed;
}
}
} else if (letter == "bksp") {
typed.pop();
typed = typed;
}
} else {
if (typed.length == 5) {
checkAnswer();
}
}
}
With the on:click elements written like so:
<div class="letter-row letter-row-a">
{#each rowOne as letter}
<button
id={letter}
on:click={() => clickedButton(letter)}
class="btn btn-outline-primary letter"
>
{letter}
</button>
{/each}
</div>
<div class="letter-row letter-row-b">
{#each rowTwo as letter}
<button
id={letter}
on:click={() => clickedButton(letter)}
class="btn btn-outline-primary letter"
>
{letter}
</button>
{/each}
</div>
<div class="letter-row letter-row-c">
{#each rowThree as letter}
<button
id={letter}
on:click={() => clickedButton(letter)}
class="btn btn-outline-primary letter"
>
{letter}
</button>
{/each}
</div>